JavaScript made me rethink my queue management, limiting simultaneous async tasks
I was rewriting an application I made in C# which had a queue of tasks to do. When the application processed the queue, it would iterate…
JavaScript, limiting simultaneous async tasks
I was rewriting an application I made in C# which had a queue of tasks to do. When the application processed the queue, it would iterate of the queue and perform the task needed for that item. The queue would kick off when the user clicked submit. Until submit is clicked, nothing dealing with the queue was running. This also allowed me to limit how many async tasks were running at the same time because I could check how many Tasks were running, sleep, and continue when there’s a slot available. Well, this didn’t work how I designed my C# application when I started writing in JavaScript.
What is this application
I can’t go into detail about this application; however, I can say that It’s an Electron application that searches some databases and APIs for names or patterns provided, either singular or list. Then find details about that name or pattern in a collection of databases and APIs. The older application is a C# WPF application that does this very thing, but is fully functional and stable, also written by me.
No Pausing or Sleep
I have some experience with writing in JavaScript, and dealing with asynchronous promises; however, this was the first time I needed to write something that required a loop to pause so that I wasn’t hitting a loop so hard the application locked up. Initially, I thought I could iterate over a list of tasks, and have a while loop that continued to execute while I’m at my maximum async tasks. This lead to the application crashing. In C#, I have a similar setup; however, the loop uses a Thread.Sleep function. This setup won’t work in JavaScript, so I needed a new method of handling a que of tasks.
Don’t Sleep, use events
For those of you that don’t know, JavaScript has events you can use. With that in mind, I can utilize the events in JavaScript to execute a block of code. Time, in JavaScript, is a condition for an event, meaning, I can have an event wait for N time to pass, then execute a function. So, I made a Que Process Manager class that would, after N time, execute a function that would iterate over all the tasks in the que. As the tasks are executed, those tasks would be marked as started. When the tasks are done, they mark themselves as done. If there’s no more slots available, meaning started/running tasks are equal to or greater than maxTasks, the loop exits. If the Que Process Manager finds tasks that have marked themselves as completed, those tasks are removed without incriminating the counter.
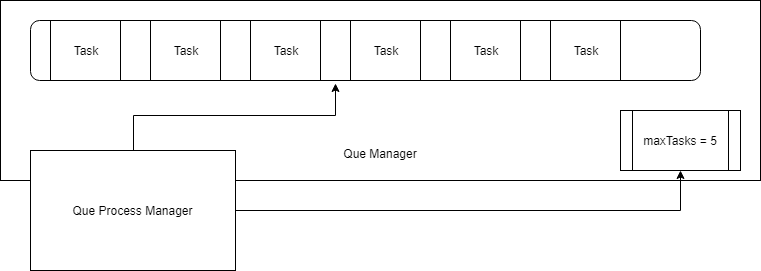
With this new method of managing my que, there’s no completed, the que is infinite. Tasks are added, and completed tasks are removed. Tasks are generic, in that they can be a request to search for something, or retrieve information about something that was found. I made it generic enough so that arbitrary tasks could be added and the Que Manager doesn’t care, it does one job.